Introduction
If you're a parent to daughters you may know what I mean when I talk about obsessions with Unicorns. When we walk pass any book or toy featuring unicorns the our girls' eyes light up with that mandatory question, "can we buy that?". My response is "No!". Please, no more, no, N-O, No!
This blog is really about TextInput validators:
- InputMask
- IntValidator
- DoubleValidator
- RegExpValidator
- InputValidator (in this example, we will show you how to say "No" to unicorns!)
The first 4 is from Qt's runtime. The last is from AppStudio's AppFramework.
InputMask
TextField {
inputMask: "999-AAA"
placeholderText: qsTr("Type in a license plate of the pattern 999-AAA")
text: "123-XYZ"
color: acceptableInput ? "green" : "red"
selectByMouse: true
}
View full InputMaskTest.qml on GitHub Gist.

InputMask is a property on TextInput components such as TextField. It allows us to quickly define acceptable text input. In the above example we allow 6 character license plates that begin with 3 digits, followed by a dash and finishing with 3 characters. When acceptable input is entered the text field changes from "red" to "green" and the user can submit the input.
The InputMask has the following features:
- When the field is "empty" you'll see placeholders for symbols like hyphen ('-'), you will not see the placeholder text
- When you type, you do not need to type the fixed symbols, e.g. hyphen ('-')
- The code is very short
https://doc.qt.io/qt-5/qml-qtquick-textinput.html#inputMask-prop
https://doc.qt.io/qt-5/qlineedit.html#inputMask-prop
IntValidator
TextField {
validator: IntValidator {
bottom: 25
top: 75
}
placeholderText: qsTr("Type in an integer between 25 and 75")
text: "34"
color: acceptableInput ? "green" : "red"
selectByMouse: true
}
View full IntValidatorTest.qml on GitHub Gist.
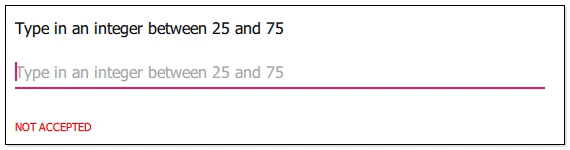
IntValidator is a QML component that can be assigned as a TextInput validator. It allows us to quickly define acceptable numerical input. In above example we allow any integer between 25 and 75. When acceptable input is entered the text field changes from "red" to "green" and the user can submit the input.
The IntValidator has the following features:
- When the field is empty you can see the placeholder text
- You cannot type more than the number of characters hinted by the IntValidator (e.g. in the above example you cannot type more than two digits)
https://doc.qt.io/qt-5/qml-qtquick-intvalidator.html
DoubleValidator
TextField {
validator: DoubleValidator {
bottom: 25.0
top: 75.0
}
placeholderText: qsTr("Type in a number between 25.0 and 75.0")
text: "34.5"
color: acceptableInput ? "green" : "red"
selectByMouse: true
}
View full DoubleValidatorTest.qml on GitHub Gist.

DoubleValidator is a QML component that can be assigned as a TextInput validator. It allows us to quickly define acceptable numerical input. In above example we allow any number between 25.0 and 75.0. When acceptable input is entered the text field changes from "red" to "green" and the user can submit the input.
The DoubleValidator has the following features:
- When the field is empty you'll see the placeholder text
- You can type in any length (because decimal points are allowed)
https://doc.qt.io/qt-5/qml-qtquick-doublevalidator.html
RegExpValidator
TextField {
validator: RegExpValidator {
regExp: /[0-9]{3}-[A-Za-z]{3}/
}
placeholderText: qsTr("Type in a license plate of the pattern 999-AAA")
text: "123-XYZ"
color: acceptableInput ? "green" : "red"
selectByMouse: true
}
View full RegExpValidatorTest.qml on GitHub Gist.

RegExpValidator is a QML component that can be assigned as a TextInput validator. It allows us to quickly define acceptable input. In the above example, we allow for license plates that begin with a 3 digit number, followed by a dash and ending with 3 letters.
RegExpValidator has the following features:
- When the field is empty you'll see the placeholder text (different than InputMask)
- You must type the hyphen, it won't be automatically typed for you (different than InputMask)
- The regular expression is significantly more complex to implement and maintain
https://doc.qt.io/qt-5/qml-qtquick-regexpvalidator.html
InputValidator
TextField {
validator: InputValidator {
validate: function (input, position) {
if (input.match(/unicorn/)) {
return InputValidator.Invalid
}
while (input.match(/canine/)) {
input = input.replace(/canine/, 'dog')
position -= 6
position += 3
}
let state = InputValidator.Intermediate
if (input.endsWith('.')) {
state = InputValidator.Acceptable
}
return { input, position, state }
}
}
placeholderText: qsTr("Type in a sentence ending with a fullstop. Avoid using 'unicorn' and 'canine'!")
text: "A dog is man's best friend."
selectByMouse: true
}
View full InputValidatorTest.qml on GitHub Gist.
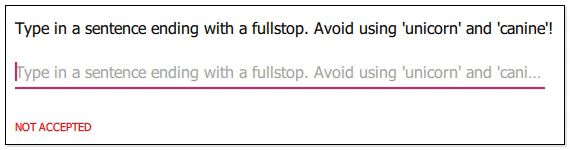
InputValidator is a QML component that can be assigned as a TextInput validator. It allows us to supply a Javascript function to be the validator. The Javascript function receives both the original string and position as input. It can return an input state (InputValidator.Invalid, InputValidator.Intermediate or InputValidator.Acceptable). It can also return an object that contains an updated version of the string, position and state. Returning an updated string allows us to implement auto correction. In the above example we forbid the user from typing in "unicorn". It's not allowed! We also forbid the user from typing "canine". If they attempt to type "canine" it gets auto corrected as "dog". The sentence will never be full accepted until the user finishes it with a full stop ".".
InputValidator has the following features:
- When the field is empty you'll see the placeholder text
- You can prohibit certain input (e.g. we can come up with a word blacklist, e.g. censor offensive words)
- You can correct / fix input (i.e. implement auto correct)
- You have full control over the experience
- Takes more effort to implement your rules
https://doc.arcgis.com/en/appstudio/api/reference/framework/qml-arcgis-appframework-inputvalidator/
References
Send us your feedback to appstudiofeedback@esri.com
Become an AppStudio for ArcGIS developer! Watch this video on how to sign up for a free trial.
Follow us on Twitter @AppStudioArcGIS to keep up-to-date on the latest information and let us know about your creations built using AppStudio to be featured in the AppStudio Showcase.
The AppStudio team periodically hosts workshops and webinars; please click on this link to leave your email if you are interested in information regarding AppStudio events.