Hi
I'm trying to develop a custom collection task for ArcGIS Mobile (10.2.1) which is based upon the SimpleDataCollectionExtension sample (code below). The collection is working fine and I can see the newly created feature on the map and it appears under 'Manage Edits'. However, when exiting the application I get this message:
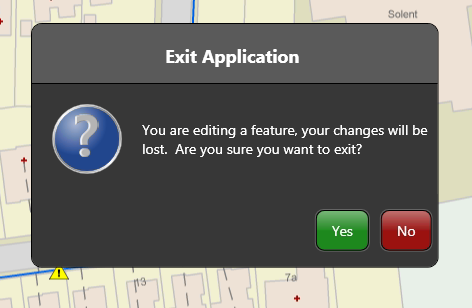
This seems to suggest that the application still thinks it's in some kind of editing mode, despite having done the following when the user clicks the OK button on the page:
geometryCollectionMethod.StopGeometryCollection();
geometryCollectionMethod.CompleteCollection();
feature.SaveEdits();
feature.StopEditing();
If I do indeed click 'Yes' to exit the application and the feature is still present when next opening the application, so the changes have been saved.
Does anyone know what I need to do to prevent the above message appearing when the application exits? What am I missing?
Regards
John
Code for the sample is:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using ESRI.ArcGIS.Mobile.Client;
using ESRI.ArcGIS.Mobile.Client.Tasks.ViewMap;
using System.Windows;
using System.Windows.Forms;
using ESRI.ArcGIS.Mobile.Client.Pages;
using ESRI.ArcGIS.Mobile.Client.Tasks.CollectFeatures;
using ESRI.ArcGIS.Mobile.FeatureCaching;
using ESRI.ArcGIS.Mobile.Client.Controls;
namespace CustomizationSamples
{
//This sample shows how to customize data collection workflows for the Windows Application.
//In View Map page, the extension adds a new button. By clicking the it, the application will jump to the geometry colltion page for the specified the layer.
public class SimpleDataCollectionExtension : ProjectExtension
{
//define the button name and layer name for customized data collection
string ButtonName = "Geometry";
string LayerName = "New Subtype";
private IPage _homePage;
private Feature _feature;
private FeatureType _featureType;
private EditFeatureAttributesPage _editFeatureAttributesPage;
private EditFeatureAttributesViewModel _editFeatureAttributesViewModel;
protected override void Initialize()
{
}
protected override void OnOwnerInitialized()
{
if (!MobileApplication.Current.Dispatcher.CheckAccess())
{
MobileApplication.Current.Dispatcher.BeginInvoke((System.Threading.ThreadStart)delegate()
{
OnOwnerInitialized();
});
return;
}
// Add three new custom commands to the View Map page to collect
// manageable areas, fire edge, and fire events.
ViewMapTask viewMapTask = MobileApplication.Current.FindTask(typeof(ViewMapTask)) as ViewMapTask;
if (viewMapTask != null)
{
//***********************************************************
// replace the layer name here
//***********************************************************
viewMapTask.ViewMapPage.ForwardCommands.Add(new UICommand(ButtonName,
param => this.CollectFireDataCommandExecute(LayerName)));
//***************************************************************
}
}
protected override void Uninitialize()
{
_editFeatureAttributesPage.ClickOk -= new EventHandler(_editFeatureAttributesPage_ClickOk);
_editFeatureAttributesPage.ClickCancel -= new EventHandler(_editFeatureAttributesPage_ClickCancel);
_editFeatureAttributesViewModel = null;
_editFeatureAttributesPage = null;
}
private void CollectFireDataCommandExecute(string featureTypeName)
{
try
{
Cursor.Current = Cursors.WaitCursor;
// Cache homepage for the application
// Once data collection is done, we come back to this cached page
_homePage = MobileApplication.Current.CurrentPage;
// Reset _feature and _featureType
_feature = null;
_featureType = FindFeatureTypeByName(featureTypeName);
if (_featureType == null)
{
ESRI.ArcGIS.Mobile.Client.Windows.MessageBox.ShowDialog("Can't find " + featureTypeName + ".", "Warning");
return;
}
// Create a new Feature, this will automatically call StartEditing on this feature
_feature = new Feature(_featureType, null);
_editFeatureAttributesViewModel = new EditFeatureAttributesViewModel(_feature);
_editFeatureAttributesPage = new EditFeatureAttributesPage(_editFeatureAttributesViewModel);
_editFeatureAttributesPage.ClickOk += new EventHandler(_editFeatureAttributesPage_ClickOk);
_editFeatureAttributesPage.ClickCancel += new EventHandler(_editFeatureAttributesPage_ClickCancel);
MobileApplication.Current.Transition(_editFeatureAttributesPage);
}
finally
{
Cursor.Current = Cursors.Default;
}
}
private FeatureType FindFeatureTypeByName(string name)
{
foreach (FeatureSourceInfo fsinfo in MobileApplication.Current.Project.EnumerateFeatureSourceInfos())
{
foreach (FeatureType featureType in fsinfo.FeatureTypes)
{
if (featureType.Name.ToLower() == name.ToLower())
return featureType;
}
}
return null;
}
void _editFeatureAttributesPage_ClickOk(object sender, EventArgs e)
{
// Save the feature
if (!SaveEdits())
ESRI.ArcGIS.Mobile.Client.Windows.MessageBox.ShowDialog("Cannot save edits.", "Warning");
// Go back to homepage
MobileApplication.Current.Transition(_homePage);
}
void _editFeatureAttributesPage_ClickCancel(object sender, EventArgs e)
{
// Cancel the edits
CancelDataCollection();
// Go back to homepage
MobileApplication.Current.Transition(_homePage);
}
private void CancelDataCollection()
{
if (_feature != null)
{
_feature.CancelEdit();
_feature.StopEditing();
}
_feature = null;
_featureType = null;
}
bool SaveEdits()
{
GeometryCollectionMethod method = _editFeatureAttributesViewModel.GeometryCollectionViewModel.GetCollectionMethodInProgress();
if (method != null)
method.StopGeometryCollection();
if (_feature != null)
{
// In case Geometry is invalid
if (_feature.Geometry == null || !_feature.Geometry.IsValid)
return false;
_feature.SaveEdits();
_feature.StopEditing();
return true;
}
else
return false;
}
}
}