Hi,
I am a little bit confused in how to integrate the arcgis api in my project.
I want to make a query to a servicelayer after the sucess ajax response, but i dont know how to integrate the api.
I am using react and reactdom, node, webpack, gulp, and I already installed the api in the server with the instructions provided by esri.
Can somebody guides me providing any example?
Thanks in advice 
My working directory is:
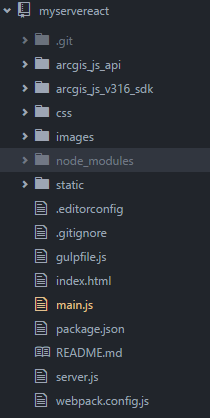
The code in my main.js file is:
import React from 'react';
import ReactDOM from 'react-dom';
function genericLogin(genericAccount, username, password){
var url = "myarcgis/arcgis/tokens/generateToken";
console.log(genericAccount);
console.log(username, password);
//Part 1, accessing with a generic account to get the token
jQuery.ajax({
type: 'POST',
url: url,
data: {
username: genericAccount.username,
password: genericAccount.password,
client: genericAccount.client,
format: 'json'
},
success: (success) => {
//Part 2: when u get the token, make a query to the service layer and see if the user who tries to log in has access , if has it - >go to the next page.
console.log("Success
: The generic account is working, verifying the user permissions... " );
console.log(success);
},
error: (error) => {
console.log("Error
: The main account is having some issue, please notify to the administrator ");
}
});
console.log('done');
}
class LoginApp extends React.Component {
constructor(){
super();
this.onClick = this.onClick.bind(this);
}
onClick(){
var genericAccount = {
username: 'myusername',
password:'mypass',
client: 'requestip'
};
var userValue = this.refs.username.value;
var passValue = this.refs.password.value;
genericLogin(genericAccount, userValue, passValue);
}
render(){
return (
<div className="wrapper">
<img className="logo" src="images/mylogo.png" />
<div className="login">
<input className="login__input" ref="username" type="text" />
<input className="login__input" ref="password" type="password" />
<input className="login__submit" type="submit" onClick={this.onClick} />
</div>
<div className="footer">
<img className="image-chq" src="images/chq_i.png" />
<p className="footer__p">some info</p>
<p className="footer__p">more info</p>
<h6 className="footer__h6">address</h6>
</div>
</div>
);
}
}
ReactDOM.render(<LoginApp />, document.getElementById('myLogin'));
My index.html
<!DOCTYPE HTML>
<html lang="es">
<head>
<meta charset="utf-8">
<title>React JSAPI</title>
<meta name="viewport" content="width=device-width, initial-scale=1, maximum-scale=1, user-scalable=no" />
<link rel="stylesheet" type="text/css" href="arcgis_js_api/library/3.16/3.16/dijit/themes/tundra/tundra.css"/>
<link rel="stylesheet" type="text/css" href="arcgis_js_api/library/3.16/3.16/esri/css/esri.css" />
<link rel="stylesheet" type="text/css" href="css/login.css"/>
</head>
<body>
<section id="myLogin"></section>
<script type="text/javascript" src="arcgis_js_api/library/3.16/3.16/init.js"></script>
<script src="static/js/vendor/jquery-2.2.1.min.js"></script>
<script src="bundle.js"></script>
</body>
</html>